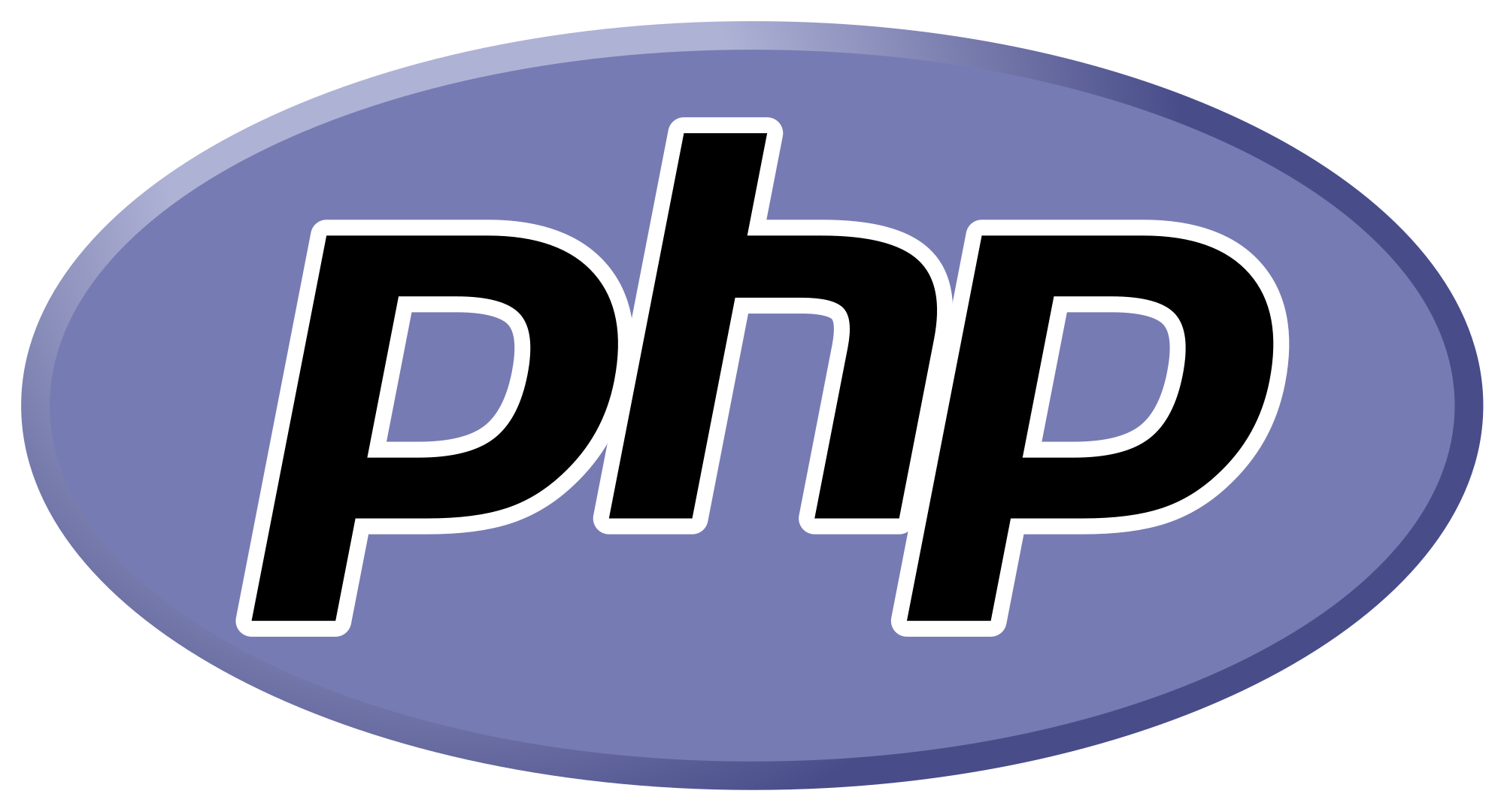
Variables can store data of different types, and different data types can do different things. Data types specify the size and type of values that can be stored. PHP is a Loosely Typed Language so here no need to define the data type.
- To check only data type use gettype( ) function.
- To check value, data type and size use var_dump( ) function.
Example
<?php $num=100; $fnum=100.0; $str="Hello"; var_dump($num,$fnum,$str); ?>
Output: int(100) float(100) string(5) “Hello”
In the above example declare three variable $num , $fnum , $str.
$num hold value=”100″. (contain integer value).
$fnum hold value=100.0 (contain float value).
$str hold value=”Hello” (contain string value).
PHP supports the following data types
- Scalar(predefined)
- Integer
- Float (floating point numbers – also called double)
- String
- Boolean
- Compound(user-defined)
- Array
- Object
- Special type
- NULL
- Resource
Integer Data Type
An integer data type is a non-decimal number between -2,147,483,648 and 2,147,483,647.
Rules for integers
- An integer must have at least one digit
- An integer must not have a decimal point
- An integer can be either positive or negative
- Integers can be specified in three formats: decimal (10-based), hexadecimal (16-based – prefixed with 0x) or octal (8-based – prefixed with 0)
In the following example, $x is an integer. The PHP var_dump() function returns the data type and value:
Example
<?php $x = 5985; var_dump($x); ?>
Output: int(5985)
Float Data Type
A float (floating point number) is a number with a decimal point or a number in exponential form.
In the following example, $x is a float. The PHP var_dump() function returns the data type and value:
Example
<?php $x = 10.365; var_dump($x); ?>
Output: float(10.365)
String Data Type
A string is a sequence of characters, like “Hello world!”. A string can be any text inside quotes. You can use single or double quotes
Example
<?php $x = "Hello world!"; $y = 'Hello world!'; echo $x; echo "<br>"; echo $y; ?>
Output:
Hello world!
Hello world!
Boolean Data Type
Boolean is the simplest data type. Like a switch that has only two states ON means true(1) and OFF means false(0).
Example
<?php $true=true; $false=false; var_dump($true,$false); ?>
Output: bool(true) bool(false)
Array Data Type
An array stores multiple values in one single variable. In the following example, $city is an array. The PHP var_dump() function returns the data type and value
Example
<?php $city = array("Chandigarh","Toronto","Boston"); var_dump($cars); ?>
Output: array(3) { [0]=> string(10) “Chandigarh” [1]=> string(7) “Toronto” [2]=> string(6) “Boston” }
Object Data Type
An object is a data type which stores data and information on how to process that data. In PHP, an object must be explicitly declared.
First, we must declare a class of object. For this, we use the class keyword. A class is a structure that can contain properties and methods
Example
<?php class Car { function Car() { $this->model = "VW"; } } // create an object $herbie = new Car(); // show object properties echo $herbie->model; ?>
Output: VW
Null Data Type
Null is a special data type which can have only one value: NULL. A variable of data type NULL is a variable that has no value assigned to it.
Tip: If a variable is created without a value, it is automatically assigned a value of NULL.
Variables can also be emptied by setting the value to NULL:
Example
<?php $x = "Hello world!"; $x = null; var_dump($x); ?>
Output: NULL
Resource Data Type
The special resource type is not an actual data type. It is the storing of a reference to functions and resources external to PHP.
A common example of using the resource data type is a database call.
Example
<?php $con = mysqli_connect("localhost","root","","users"); ?>
The function will return a resource type data to be stored into $con variable.
Some Predefine functions to Check data type
- is_int( ) : Check given value is integer or not
- is_float( ) : Check given value is float or not
- is_numeric( ) : Check given value is either integer or float
- is_string( ) : Check given value is string or not
- is_bool( ) : Check given value is Boolean or not
- is_array( ) : Check given value is array or not
- is_object( ) : Check given value is object or not
- is_null( ) : Check given value is null or not
Example
Check if given variable holds a integer type of value then print the sum otherwise show error message)
<?php
$x = 1000;
$y = 500;
if(is_int($x) && is_int($y))
{
$sum = $x + $y;
echo "sum = ".$sum;
}
else
{
echo "both number must be integer";
}
?>
Output: sum = 1500
In the above example: Create two variable $x hold value=100, $y hold value=500, now execute if..else condition. We pass is_int( ) function inside if condition, to check the value is integer or not , if yes statement is execute and print the sum of two values. if not else statement is execute show an error message.